工厂方法
# 工厂方法(Factory Method)
# Intent
定义了一个创建对象的接口,但由子类决定要实例化哪个类。工厂方法把实例化操作推迟到子类。
# Class Diagram
在简单工厂中,创建对象的是另一个类,而在工厂方法中,是由子类来创建对象。
下图中,Factory 有一个 factory() 方法,这个方法需要用到一个产品对象,这个产品对象由 factoryMethod() 方法创建。该方法是抽象的,需要由子类去实现。
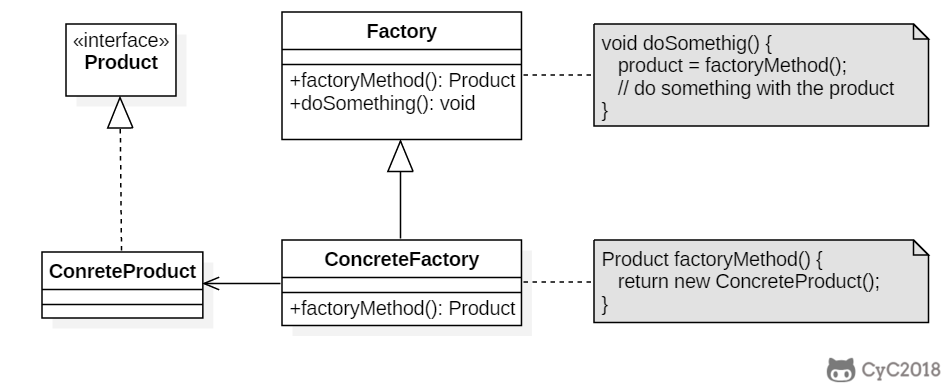
# Implementation
package com.code.factory.example;
public abstract class Product {
abstract public void onSay();
}
1
2
3
4
5
2
3
4
5
package com.code.factory.example;
public class CommonProduct extends Product {
public void onSay(){
System.out.println(toString() + " : CommonProduct");
}
}
1
2
3
4
5
6
7
2
3
4
5
6
7
package com.code.factory.example;
public class MobileProduct extends Product {
public void onSay(){
System.out.println(toString() + " : MobileProduct");
}
}
1
2
3
4
5
6
7
2
3
4
5
6
7
package com.code.factory.example;
public class ComputerProduct extends Product {
public void onSay(){
System.out.println(toString() + " : ComputerProduct");
}
}
1
2
3
4
5
6
7
2
3
4
5
6
7
package com.code.factory.example;
public abstract class Factory {
abstract protected Product factoryMethod();
public Product factory() {
return factoryMethod();
}
}
1
2
3
4
5
6
7
8
2
3
4
5
6
7
8
package com.code.factory.example;
public class MoblieFactory extends Factory {
protected Product factoryMethod() {
return new MobileProduct();
}
}
1
2
3
4
5
6
7
2
3
4
5
6
7
package com.code.factory.example;
public class ComputerFactory extends Factory {
protected Product factoryMethod() {
return new ComputerProduct();
}
}
1
2
3
4
5
6
7
2
3
4
5
6
7
package com.code.factory.example;
public class CommonFactory extends Factory {
protected Product factoryMethod() {
return new CommonProduct();
}
}
1
2
3
4
5
6
7
2
3
4
5
6
7
测试例子
package com.code.factory.example;
public class Main {
public static void main(String[] args) {
Factory factory;
factory = new CommonFactory();
Product commonFactory = factory.factory();
commonFactory.onSay();
factory = new MoblieFactory();
Product mobileFactory = factory.factory();
mobileFactory.onSay();
factory = new ComputerFactory();
Product computerFactory = factory.factory();
computerFactory.onSay();
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
com.code.factory.example.CommonProduct@123a439b : CommonProduct
com.code.factory.example.MobileProduct@3c679bde : MobileProduct
com.code.factory.example.ComputerProduct@16b4a017 : ComputerProduct
1
2
3
2
3
# JDK
- java.util.Calendar (opens new window)
- java.util.ResourceBundle (opens new window)
- java.text.NumberFormat (opens new window)
- java.nio.charset.Charset (opens new window)
- java.net.URLStreamHandlerFactory (opens new window)
- java.util.EnumSet (opens new window)
- javax.xml.bind.JAXBContext (opens new window)